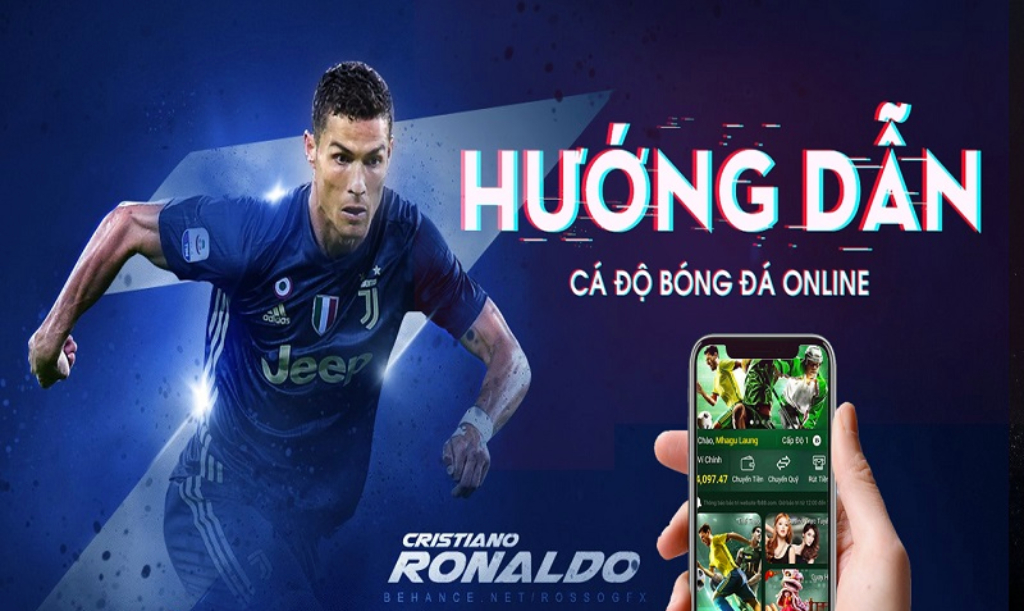
Tập trung vào ngành công nghiệp trò chơi xổ số trong nhiều năm, với màu sắc và cách chơi cổ điển. Ngoài ra còn có rất nhiều trò chơi độc quyền và sáng tạo, và giao diện trò chơi đủ mới lạ và dễ vận hành, điều này sẽ tạo thêm vẻ bóng bẩy cho quá trình chơi game của bạn!
1、QUÉT MÃ QR BẰNG ĐIỆN THOẠI DI ĐỘNG CỦA BẠN, NHẬP TRANG TẢI XUỐNG APP VÀ NHẤP ĐỂ TẢI XUỐNG。
2、VUI LÒNG LÀM THEO HƯỚNG DẪN TRÊN TRANG NÀY ĐỂ CÀI ĐẶT VÀ ỦY QUYỀN APP。
Tìm kiếm nhiều: #123b, #casino 123b, #nhà cái 123b, #link vào 123b, #tại 123b, #trang chủ 123b #123bbet #123bigo #123bia # 123b đăng nhập # 123b khuyến mãi 100k #123b đăng nhập